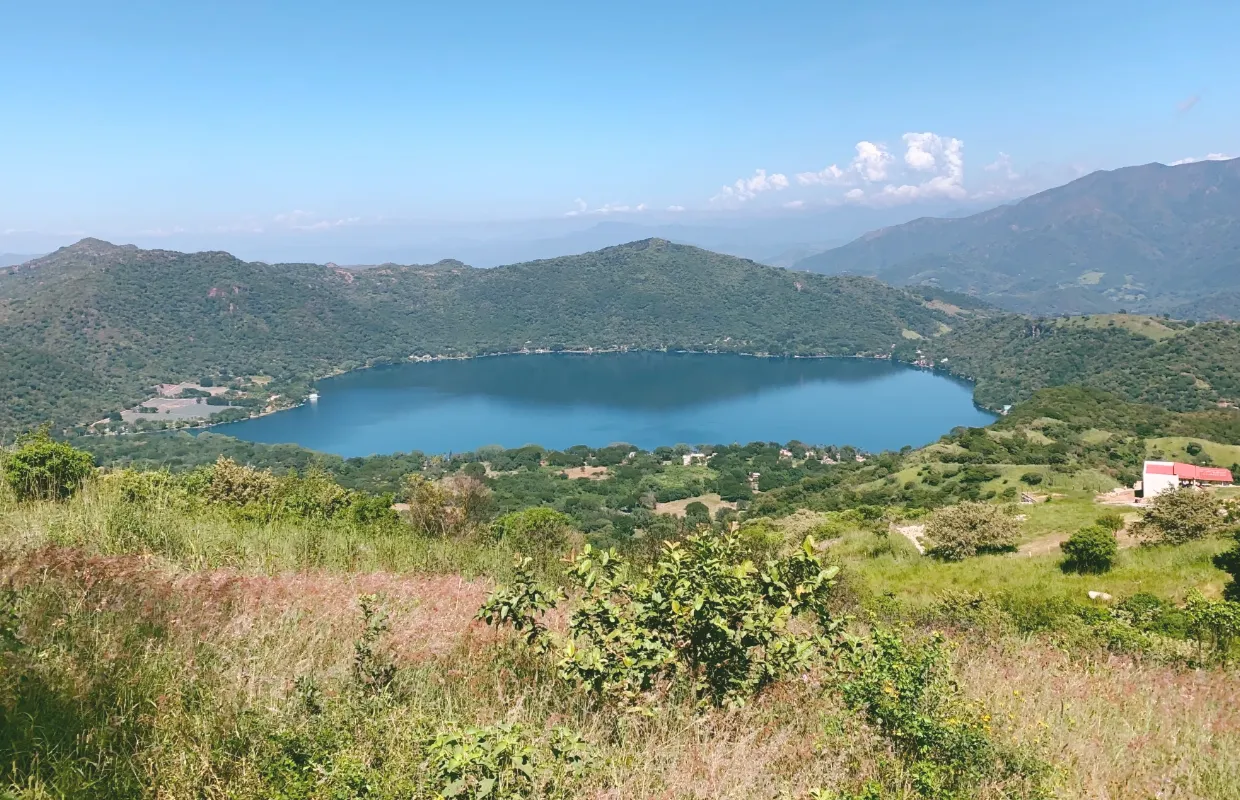
Monday, August 9 2021
Accepting SvelteKit hooks and Fastify
Accepting SvelteKit hooks and Fastify
SvelteKit has been in beta for a while and I can see the potential of building progressively enhanced websites with it, but one of my main issues is that I don’t want to run a different service for the APIs I’m used to build using fastify.
I’ve tried several things in my experiments of trying to combine fastify and svelte, but in the end it’s all about learning to accept SvelteKit adapters and use the hooks provided to accomplish the necessary integration.
Getting Started
SvelteKit comes with a node adapter we can use. By using the hooks handler we will intercept the requests and decide whether it should by handle by SvelteKit or if it should be handled by fastify.
Let’s start by creating a new SvelteKit project.
# create your project
npm init svelte@next my-app
cd my-app
Now let’s install fastify and the necessary svelte kit dependencies.
npm install -D @sveltejs/adapter-node@next fastify
We will have to modify the svelte.config.js
file in order to use the adapter-node
package.
import node from '@sveltejs/adapter-node';
/** @type {import('@sveltejs/kit').Config} */
const config = {
kit: {
// hydrate the <div id="svelte"> element in src/app.html
target: '#svelte',
adapter: node()
}
};
export default config;
Setup Fastify
To get SvelteKit and fastify to communicate we need to export a fastify instance that will be used inside of the hook
// src/server.js
import Fastify from 'fastify'
export async function init () {
const fastify = Fastify({});
fastify.get('/api/health', (request, reply) => {
reply.send({ status: 'ok' })
});
// register any extra routes here
fastify.register(await import('./plugins/users'), { prefix: '/api' });
return fastify;
}
The init
function creates a new fastify instance but does not starts listening for requests, that should be handled by the SvelteKit hook.
// src/hooks.js
import * as Server from './server'
export async function handle({ request, resolve }) {
// route all requests to fastify if they have the /api prefix
if (request.path.startsWith('/api')) {
const server = await Server.init();
const response = await server.inject({
method: 'GET',
url: request.path,
query: request.query,
payload: request.payload,
headers: request.headers
});
// return fastify's response with a request object SvelteKit can understand
return {
status: response.statusCode,
body: response.body
}
}
const response = await resolve(request);
return {
...response,
headers: {
...response.headers,
// add any extra headers here
'x-custom': 'hi'
}
}
}
Fastify supports injecting requests into the server instance which can be used when creating unit tests for your routes, but it’s also used by the official serverless plugin aws-lambda-fastify, which means it should be allowed to be used in production.
Conclusion
This should be enough for running fastify and SvelteKit in your applications, though the only downside might be that the node server will be combined in a giant js file, which might make it harder to debug any server issues.
If you want to use fastify for the entire request lifecycle, maybe a solution like fastify-vite will work out for you. There’s no svelte examples in the repository but svelte already has an official vite plugin you can use. https://www.npmjs.com/package/@sveltejs/vite-plugin-svelte